Codecov
Codecov is a tool that allows us to check the code coverage. Code coverage is a measurement used to express which a test suite executed lines of code. The code coverage is calculated using three conditionals:
- Hit 🎯 — indicates that the test suite executed the source code.
- Partial 🌦 — indicates that the test suite did not fully execute the source code.
- Miss 🤷 — indicates that the test suite did not execute the source code.
To calculate, we have to apply this formula: Coverage ratio = Hits / (Hit + Partial + Miss)
For example, a codebase that has 5 lines executed by tests out of 12 total lines will receive a coverage ratio of 41% (rounding down).
What does Codecov bring to the table?
Codecov has a focus on integration and promoting healthy pull requests. It delivers coverage metrics directly into the modern workflow to promote more code coverage.
How can we connect CircleCI with Codecov?
Before connecting to CircleCI we have to configure Codecov in our GitHub project. The first step is to visit the CodeCov website and sign up with GitHub. Then connect to the desired repository and save the given token:
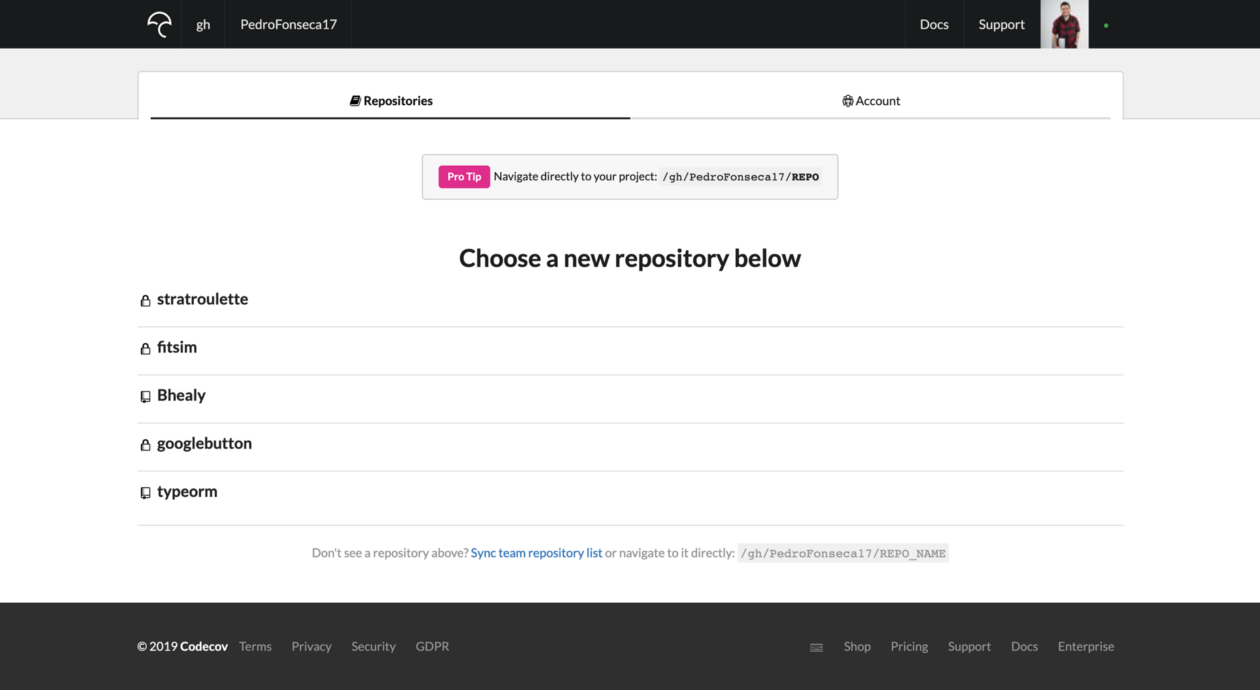
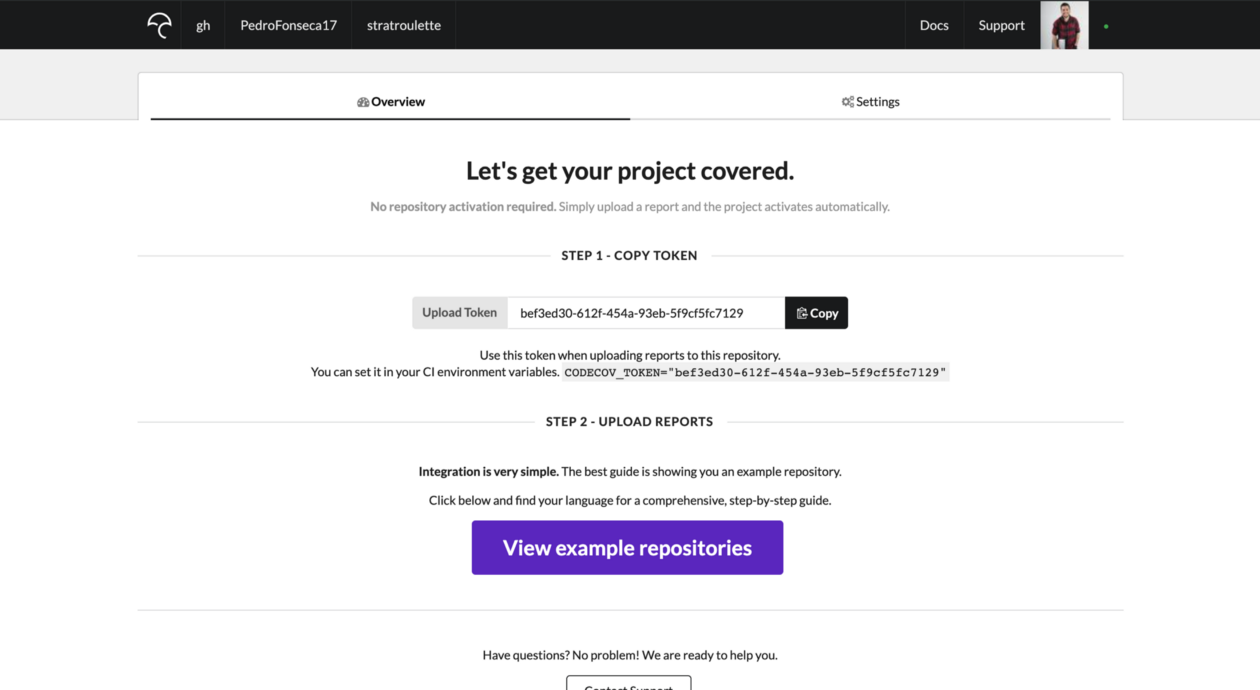
After that we need to install Codecov in the project with:
npm install -g codecov
And add this to the package.json file:
-- CODE language-json line-numbers --
"jest": {<br>
"coverageDirectory": "./coverage/",<br>
"collectCoverage": true<br>
}
The last step is running “npm test && codecov” which will create the coverage folder. Now we are ready to connect Codecov with CircleCI, and what do we need?
- Create a .codecov.yml ⚙️
- Use the codecov orb on the CircleCI config 🔮
So now, we create a .codecov.yml file on the root folder and add these lines:
-- CODE language-yml line-numbers --
codecov:<br>
require_ci_to_pass: yes<br>
coverage:<br>
precision: 2<br>
round: down<br>
range: "70...100"<br>
status:<br>
project: yes<br>
patch: yes<br>
changes: no<br>
parsers:<br>
gcov:<br>
branch_detection:<br>
conditional: yes<br>
loop: yes<br>
method: no<br>
macro: no<br>
comment:<br>
layout: "reach,diff,flags,tree"<br>
behavior: default<br>
require_changes: no<br>
With the file created there is only a step until all is configured and that is to use the Codecov orb on the CircleCI config. But what is an orb?
CircleCI Orbs
CircleCI orbs are shareable packages of configuration which will make everything easy to use and configure. Now that we’ve learned what orbs are, let’s add it to the project!
The first step is to update the CircleCI config file version to 2.1 and add the orbs we want bellow:
-- CODE language-yml line-numbers --
version: 2.1<br>
orbs:<br>
codecov: codecov/codecov@1.0.2<br>
Then, we need to insert the command “upload” from the orb and provide the file of the report and the token that Codecov generated our test job.
-- CODE language-yml line-numbers --
Run-With-Node:<br>
docker:<br>
- image: circleci/node:10-browsers<br>
steps:<br>
- checkout<br>
- run:<br>
name: install and run tests<br>
command: |<br>
yarn install && yarn test<br>
- codecov/upload:<br>
file: './coverage/clover.xml'<br>
token: a7a5df63-codcov-token-4278vh38<br>
I know I said that this was the final step, but actually, there is one more 🙄
Certified vs. 3rd-Party Orb
CircleCI has available some individual orbs that have been tested and certified to work with the platform while all other orbs are considered 3rd-party orbs.
These 3rd-party orbs need an extra step and as you have already guessed Codecov orb is a 3rd-Party Orb.
What should we do?
The solution is simple: we just need to go to the security projects of our account or team and “Allow uncertified orbs”.

Codecov orb is set! 🥳
Now it is time to a new status check on the branch protection and add a friendly report bot. We add the status check by accessing again to the projects settings as we did before and add the new tick.

To add the friendly report bot, we need to access the Codecov app page and press the configure button.
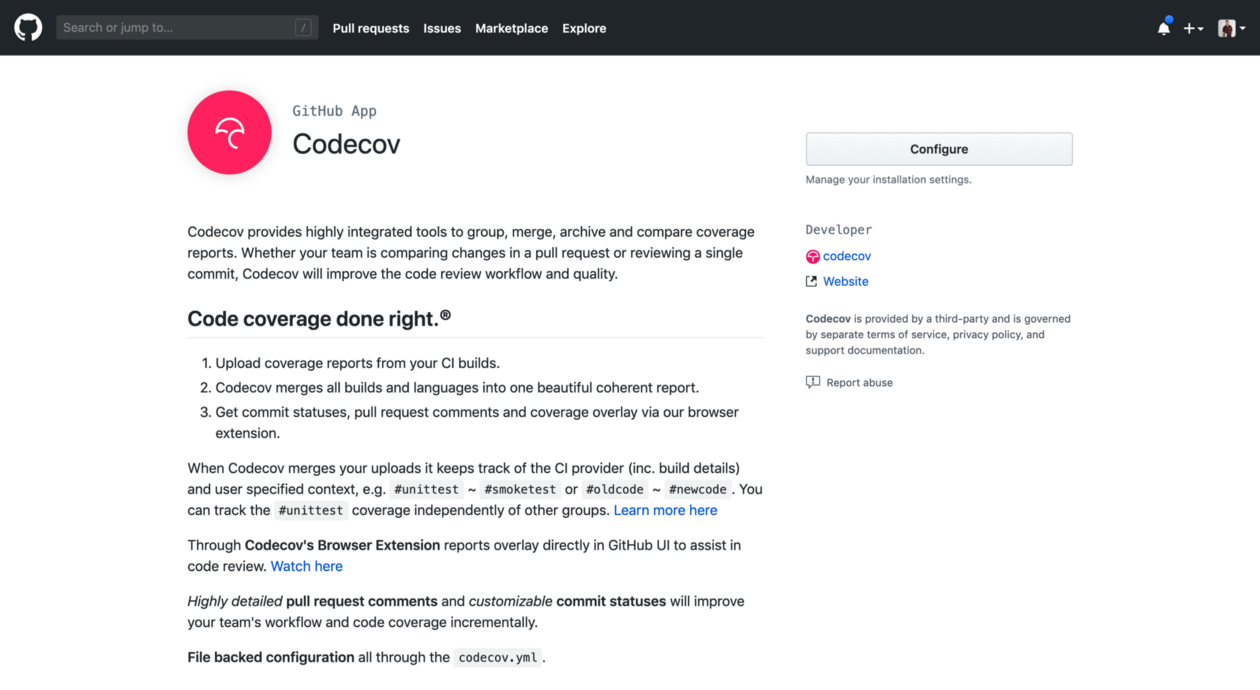
Then select the account and install the Codecov bot.

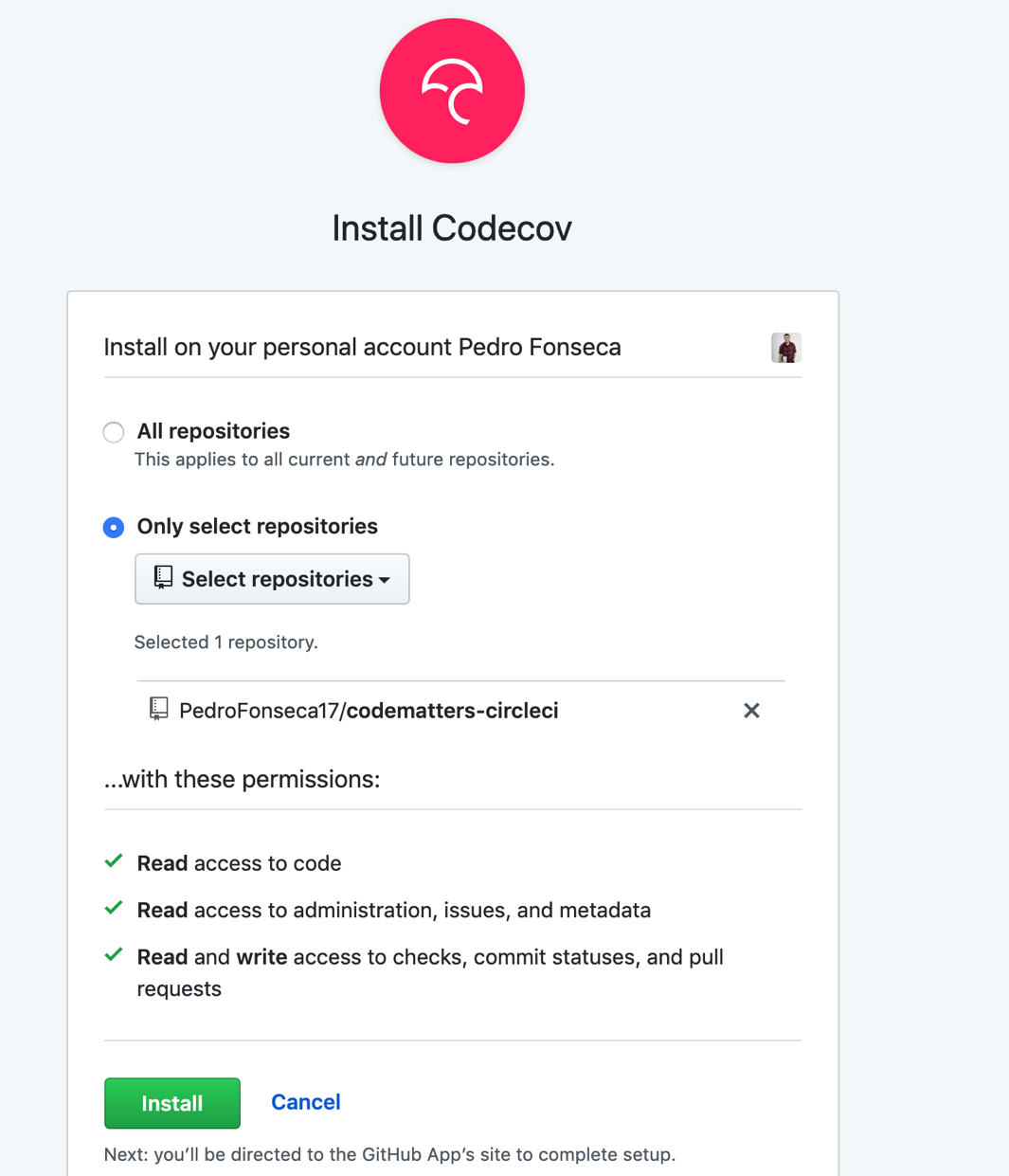
The robot is activated! 🚀
These are some reports that the robot will make:

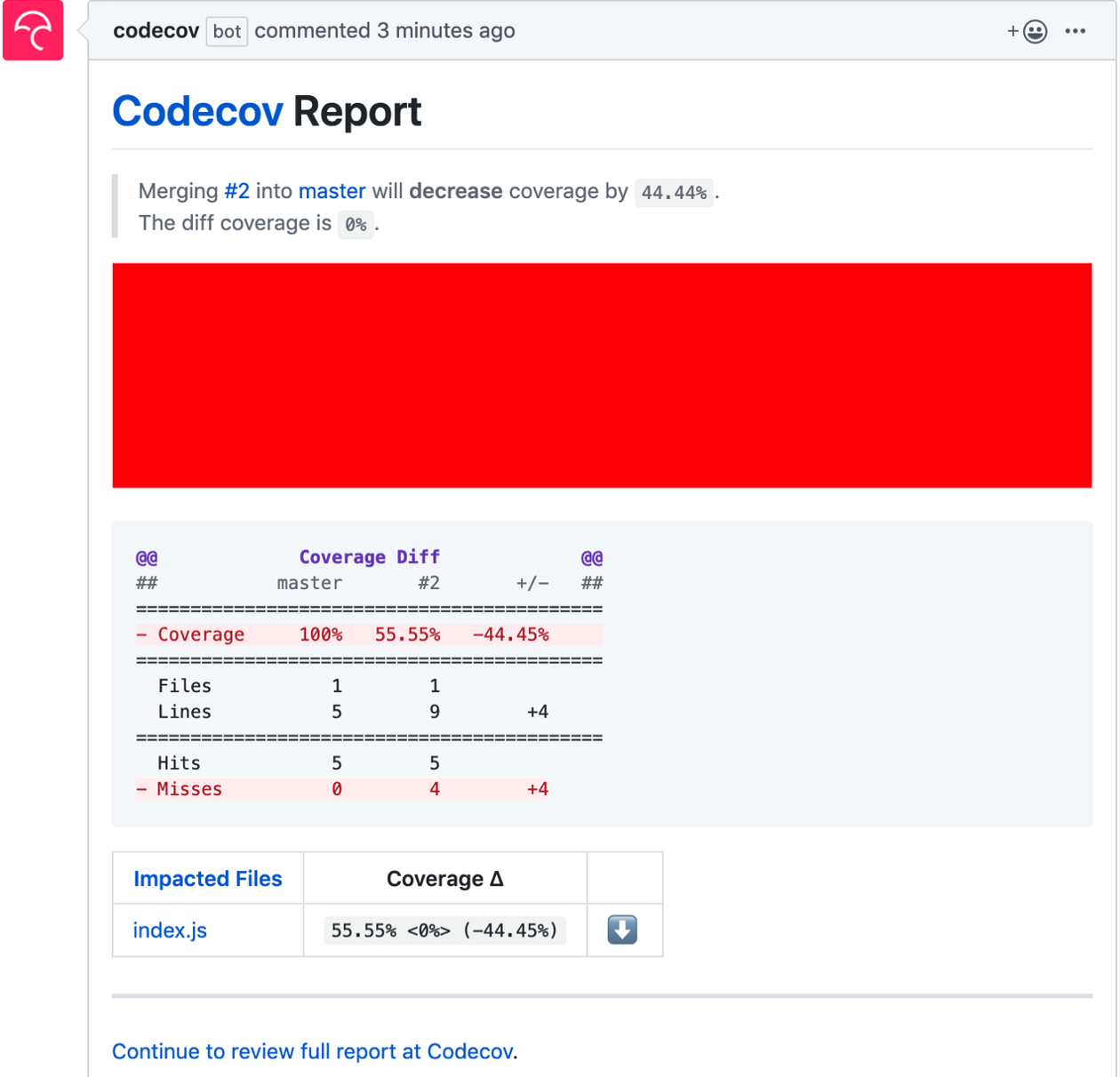

All is done! Now you have CircleCI and CodeCov in your project. 🎉 🥳
The final version of the code can be accessed here. Feel free to clone it and use it in your projects, just don’t forget to replace the codecov upload token with yours!