If you're eager to master the art of creating top-notch API endpoints, you're in the right place.
In this article, we'll cover the basics of RESTful API endpoints, best practices for security, error handling, scalability, and more, with a special focus on the importance of secure traffic, limiting the rate of requests, validating inputs, filtering out unnecessary information, and proper error handling.
Don't worry. We haven't forgotten the importance of scalability. We'll discuss how to design your architecture with scalability in mind so you have all the right ingredients to create API endpoints the right way.
What is an API Endpoint?
Before we start creating API endpoints, let's take a moment to understand what an API endpoint is and what its purpose is.
An API endpoint is a specific URL that we use to make requests to an API that generates a response. When creating API endpoints, it's important to ensure, firstly, that they are secure and well-documented. Secondly, we should ensure that the parameters and data sent in requests are valid. Additionally, we should consider adding versioning and caching mechanisms to improve performance and data integrity.
By following best practices in areas such as security, performance, and error handling, we can create endpoints that are secure, performant, and user-friendly.
5 Best practices for designing new API endpoints
Let's explore some best practices to keep in mind when designing new API endpoints that are secure, high-performing, and user-friendly.
One keyword to keep in mind when creating new endpoints is "security". Security is essential when creating endpoints because the business relies on it - this is reason enough to be concerned and take it seriously.
If our infrastructure suffers a security breach, there are several consequences, such as:
- Hours of downtime to resolve these security breaches can result in the loss of clients while everything is down.
- Loss of reputation and compliance issues.
- Higher infrastructure bills due to possible API key compromise.
These are just a few reasons why we should always think about security. But how do we approach it? 🤔 Here are 5 best practices you should always follow.
1. Secure traffic
It is highly recommended that all network traffic be encrypted to ensure the security and privacy of the data being transmitted. This can be achieved by using secure protocols such as HTTPS, which relies on SSL certificates to encrypt the data being transferred between the server and the client.
The API should also require HTTPS connections only to ensure that all requests and responses are encrypted. Using an SSL certificate not only adds an additional layer of security but also helps prevent malicious attacks and unauthorized access to sensitive information.

2. Rate Limiting
Rate limiting restricts the number of requests a client can make to an API endpoint. They are normally set within a specific time frame and can be defined per user, IP address, or an API token, for example.
Using rate limiting the right way provides multiple benefits to our entire system, such as:
- Cost Management: By avoiding having multiple unwanted requests from a client, we can avoid unnecessary costs, especially when using cloud services that usually charge by use.
- Resource Protection: It prevents a single person from overloading your servers and ensures that your resources are available to all your users.
- Security: Attacks such as DDoS or brute-force login attempts can be avoided by implementing rate limiting.
To apply rate limiting, there are some useful strategies that can be applied:
- Time frame: Allow only a maximum number of requests per time frame, for example, 1000 requests per hour.
- Token bucket: The user has a set of tokens that accumulate at a set rate, and each request costs a token. If the user runs out of tokens, they need to wait to receive more.
- Leaky Bucket: It’s similar to a queue; the requests enter a bucket and are processed at a fixed rate. If the bucket overflows, the excess requests are discarded.
Sometimes a user may try to abuse the system, and in those cases, there are multiple ways to block them from making any requests at all.
- API token revocation: If the user is using a specific token to access your API, a good way to prevent access is to revoke it. This way, the user would be completely blocked unless they create a new account.
- Block IP Address: This method only blocks the specific user and is effective, but it can be easily bypassed by using a VPN and can also block legitimate users if the IP belongs to a public entity such as schools or companies.
- CAPTCHA: It’s a good way to prevent bots from making unwanted requests, but it can also negatively affect the user experience for legitimate users.
- Behavior Analysis: This method requires advanced technology to identify suspicious patterns, but it can be very effective against bots and automated attacks. However, it has the downside of possible false positives.
3. Validating inputs
A foundational step to avoid API attacks, such as SQL injection, is to validate every input that your application receives. Make sure that it comes in a valid format and that the POST request size is not too big since trying to parse a large input can consume a lot of your infrastructure resources.
There are various ways of validating your inputs, such as:
- Validation of data types: Make sure your API is receiving the exact type of data it expects. Expecting a number and receiving a string should be rejected.
- Range and Length checks: Whenever you have a piece of data that can be inside a range, you should always validate if the data received is between that range. For strings, there’s always the option to set a maximum length of characters to avoid long inputs.
- Whitelisting: If you are expecting a value that can only be one of five possible values, explicitly check if the data you are receiving is in accordance with the expected data.
Aligned with these techniques, there’s the possibility to create a Middleware. A middleware is a specific part of your code that can be used to perform various tasks, including input validation. This way, you have reusable code to validate your inputs in every place of your application.
As a last method, but not the least, you can sanitize your data to ensure that it is safe to process.
- Escape characters: Ensure that everything unwanted gets escaped. For example, special characters that could prevent SQL Injections.
- Remove unwanted data: Remove every piece of data that shouldn’t be there.
- Use prepared statements: Ensure that the data you receive is always treated as data and never as executable code.
4. Filter out unnecessary information
It is important to ensure that your API endpoint only returns the information that is necessary for the specific request. This helps prevent potential security risks and avoid unwanted data leaks. For example, minimizing exposure is a good practice because there is always data that seems harmless, but that can potentially be used by malicious actors in ways you may not anticipate.
Use security principles like the "Principle of Least Privilege", which states that a system only needs to provide the minimum access or information necessary to perform a task, reducing the risk of unwanted data leaks.
To achieve this, it is recommended to carefully analyze the requested information and identify what is actually needed for each specific request. Additionally, it may be useful to implement filters or other mechanisms to ensure that only the relevant information is returned.
5. Error Handling
When creating an API, it's important to ensure that it's user-friendly. One of the best ways to achieve this is to implement proper error handling and provide the correct status codes. Error handling is a critical aspect of API design. It's essential to handle and respond to errors effectively to ensure that users can understand what is happening when something goes wrong.
By providing appropriate status codes, the API user can quickly identify the type of error that occurred and take corrective action if necessary. The correct status codes can also help diagnose the cause of the error, making it easier to troubleshoot and fix the problem.
HTTP status codes are used to indicate the type of response returned by the server. They are divided into five categories:
- 100-level (Informational) – the server acknowledges a request.
- 200-level (Success) – the server completed the request as expected.
- 300-level (Redirection) – the client needs to perform further actions to complete the request.
- 400-level (Client error) – the client sent an invalid request.
- 500-level (Server error) – the server failed to fulfill a valid request due to an error with the server.
Each status code provides a different level of information about the response. The correct status code helps streamline the communication between the API and its users, making it easier to understand what went wrong.
Within these five categories, there are many different types of status codes that can be used to indicate specific types of errors. For example:
- 400 Bad Request – the client sent an invalid request, such as an invalid required body or parameter.
- 401 Unauthorized – the client failed to authenticate with the server.
- 403 Forbidden – the client does not have permission to access the requested resource.
- 404 Not Found – the requested resource does not exist.
- 500 Internal Server Error – a generic error occurred on the server.
- 503 Service Unavailable – the requested service is not available.
In conclusion, proper error handling and providing the correct status codes are essential for API design. They allow for a smooth use of your API and avoid unwanted frustrations, making it user-friendly.
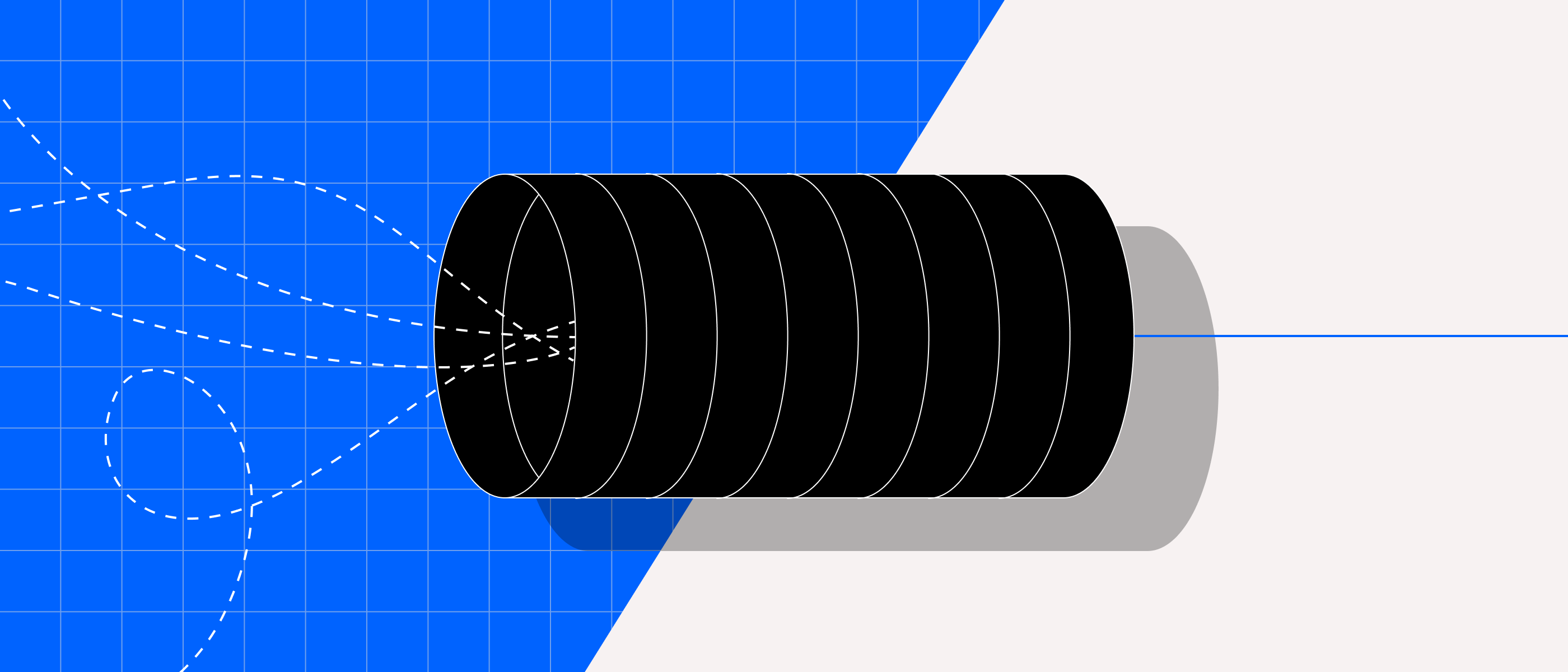
Conclusion
Creating API endpoints requires careful consideration of security, error handling, validation, and user-friendliness. By following best practices in each of these areas, API designers can create secure, high-performing, and user-friendly endpoints that meet the needs of their users.
From understanding to execution – you're all set.
Channel your newfound knowledge into crafting top-tier API endpoints!